API reference
This page documents Flatfile's REST API, focused mainly around retrieving data from Flatfile.
We are continuing to develop new endpoints based on developer feedback. Please reach out to Support to share your thoughts on how we can improve our APIs to support your needs.
Hosts
The primary host is api.us.flatfile.io
for both read and write operations. All API access must use HTTPS.
Format
The entire API uses JSON encoded as UTF-8.
The body of POST and PUT requests must be either a JSON object or a JSON array (depending on the particular endpoint) and their Content-Type
header should be set to application/json; charset=UTF-8
.
The body of responses is always a JSON object, and their content type is always application/json; charset=UTF-8
.
Managing access keys
You will need an access key to make API requests, and access keys are managed within your Flatfile account dashboard. To create an access key and start making reqests:
- Visit your Flatfile account dashboard.
- In the bottom-left corner you should see a User Profile area. Click on this area to open a menu, and select Settings.
- On the Account Settings page, under the "Account Settings" section at the bottom of the page, click the Manage access keys button. You can create new access keys and also deactivate any previously generated access keys on this page.
- Click the Create access key button. In the modal, enter a reference name in the "Memo" field and click the Create button.
- Next you will see a new modal with an "Access Key Id" and the associated "Secret Access Key"; the latter is only displayed once, so do not forget to copy it before continuting to avoid repeating this process again. This key pair grants you access to whichever API you choose. Note that access keys are assigned to your User Profile, and are not visible to other users in your team.
You can provide API Authorization via either the header: "X-Api-Key": "Access_Key_Id+Secret_Access_Key
(must include the +
sign) or "Authorization": "Bearer <token>"
.
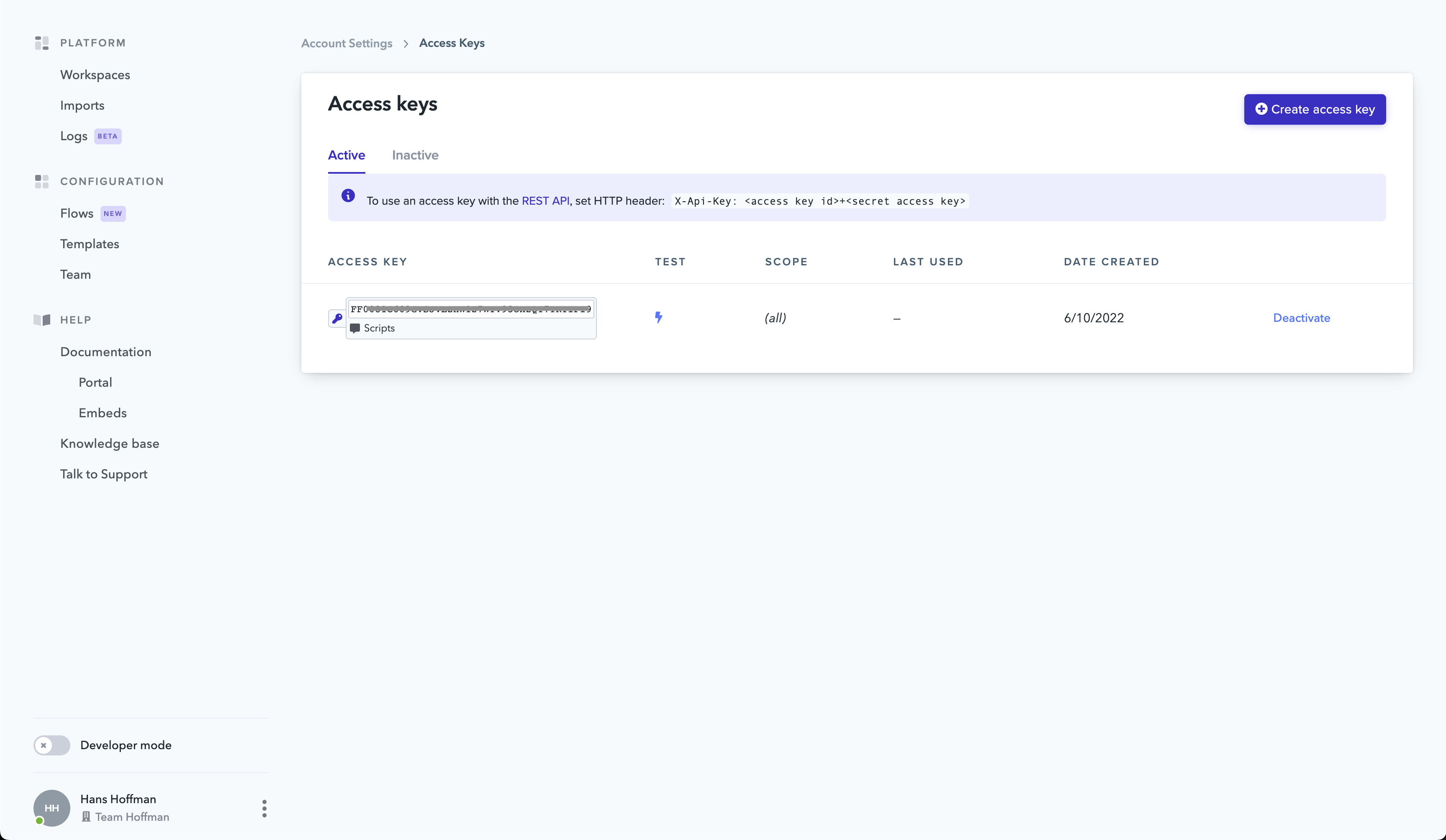
Endpoints
POST
Exchange access key for JWT
Endpoint: /auth/access-key/exchange
Request Body:
Name | Required | Type | Value | Description |
---|---|---|---|---|
accessKeyId | true | string | Access Key generated in app | |
expiresIn | number | 43200 (default) | Sets an expiration (in seconds) | |
secretAccessKey | true | string | Secret Access Key generated in app |
Example Response:
- Types
- JSON
type JWT = string;
interface Reponse {
accessToken: JWT;
user: {
id: number;
name: string;
email: string;
type: "other" | "unknown";
jobTitle: Nullable<string>;
githubUsername: Nullable<string>;
trialEndsAt: Nullable<ISO_8601>;
lastLoginAt: ISO_8601;
createdAt: ISO_8601;
updatedAt: ISO_8601;
};
}
{
"accessToken": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VybmFtZSI6ImhhbnNAZmxhdGZpbGUuaW8iLCJzdWIiOiIzMDE4MCIsImFjY2Vzc0tleUlkIjoiRkYwMDgxQzYwOUdWU0pWTFpIVzFaN1dZVjkzT1haUVQ3WUtZSVBJOSIsImlhdCI6MTY1NTc0MTg2MSwiZXhwIjoxNjU1NzQxOTIyfQ.JE-LsQ8jRCd14PHK-cd1W3cr9tpUXrIlztNJQuL5cb8",
"user": {
"id": 30180,
"name": "Foo Bar",
"email": "foobar@flatfile.io",
"type": "other",
"jobTitle": null,
"githubUsername": null,
"trialEndsAt": null,
"lastLoginAt": "2022-06-17T18:36:34.000Z",
"createdAt": "2022-05-02T16:08:12.000Z",
"updatedAt": "2022-06-17T18:36:34.000Z"
}
}
GET
Download an upload
Endpoint: /batch/:batchId/export.csv
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
batchId | true | string | A valid UUID |
Query Params:
Name | Required | Type | Value | Description |
---|---|---|---|---|
type | true | string | Enum: original , processed | File to download |
Response: Binary Data
DELETE
Delete an upload
Endpoint: /batch/:batchId
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
batchId | true | string | A valid UUID |
Example Reponse:
- Types
- JSON
interface Response extends Batch {
__schema__: Schema;
__embed__: Embed;
}
{
"id": "a4b38573-58ba-4eb0-9e2a-423044617836",
"licenseId": 30335,
"teamId": 34661,
"environmentId": "25e01f6b-08f8-4cea-ba11-892a0c0bbe59",
"workspaceId": "5409bc4c-9c10-4be1-9171-8975884d1dc5",
"schemaId": 150088,
"embedId": "285cc1c6-db43-43a9-85be-3f4e90caec5b",
"endUserId": "974ea422-8686-4de6-b17b-e454cc3e07b7",
"filename": "license-0368488c-db40-4cb4-b949-63547207b58b/batch-a4b38573-58ba-4eb0-9e2a-423044617836/upload-3e5b7648-0988-439b-89be-5a1540c07ae8.csv",
"source": null,
"memo": null,
"validatedIn": null,
"originalFile": "20+_CRM_Contacts.csv",
"manual": false,
"managed": false,
"countRows": 22,
"countRowsInvalid": null,
"countRowsAccepted": null,
"countColumns": null,
"countColumnsMatched": null,
"headersRaw": null,
"headersMatched": [
{
"index": 0,
"letter": "",
"value": "Status",
"matched_key": "dealStatus"
},
{
"index": 1,
"letter": "",
"value": "Date of Birth",
"matched_key": "date"
},
{
"index": 2,
"letter": "",
"value": "Zip",
"matched_key": "postalCode"
},
{
"index": 3,
"letter": "",
"value": "Country",
"matched_key": "country"
},
{
"index": 4,
"letter": "",
"value": "Phone",
"matched_key": "phone"
},
{
"index": 5,
"letter": "",
"value": "Subscribed",
"matched_key": "optIn"
},
{
"index": 6,
"letter": "",
"value": "Email",
"matched_key": "email"
},
{
"index": 7,
"letter": "",
"value": "last",
"matched_key": "lastName"
},
{
"index": 8,
"letter": "",
"value": "first",
"matched_key": "firstName"
}
],
"status": "submitted",
"type": 2,
"targetSchema": null,
"parsingConfig": null,
"importedFromUrl": "https://zmkcjc.csb.app/",
"headerHash": null,
"failureReason": null,
"matchedAt": null,
"submittedAt": "2022-06-22T14:31:45.000Z",
"failedAt": null,
"handledAt": null,
"writeAccessKey": "5c92379f-7405-4a14-8e33-33247425ad47",
"legacySettingsId": null,
"legacyWebhookUrl": null,
"legacyHasFieldHooks": null,
"legacyHasRecordHooks": null,
"createdAt": "2022-06-21T17:18:27.000Z",
"updatedAt": "2022-06-24T21:23:43.000Z",
"devMode": false,
"deleted": true,
"__schema__": {
"id": 150088,
"name": "CRM Contacts Demo",
"ancestorId": 149805,
"variantOfId": null,
"rootId": 139270,
"version": 111,
"archived": true,
"teamId": 34661,
"environmentId": "25e01f6b-08f8-4cea-ba11-892a0c0bbe59",
"createdById": 30180,
"jsonSchema": {
"schema": {
"properties": {
"firstName": {
"type": "string",
"label": "First Name"
},
"lastName": {
"type": "string",
"label": "Last Name"
},
"email": {
"type": "string",
"label": "Email Address",
"format": "email"
},
"phone": {
"type": "string",
"label": "Phone Number",
"format": "phone"
},
"date": {
"type": "string",
"label": "Date"
},
"country": {
"type": "string",
"label": "Country"
},
"postalCode": {
"type": "string",
"label": "Postal Code"
},
"optIn": {
"type": "boolean",
"label": "Opt In"
},
"dealStatus": {
"type": "string",
"label": "Deal Status",
"enumLabel": [
"Prospecting",
"Discovery",
"Proposal",
"Negotiation",
"Closed Won",
"Closed Lost"
],
"enum": [
"Prospecting",
"Discovery",
"Proposal",
"Negotiation",
"Closed Won",
"Closed Lost"
]
}
},
"type": "object",
"required": ["lastName"],
"unique": ["email"]
}
},
"importCount": 0,
"fieldCount": 9,
"createdAt": "2022-06-18T20:33:07.000Z",
"updatedAt": "2022-06-24T17:42:49.000Z",
"linkedSchemaIds": [],
"previewFieldKey": null
},
"__embed__": {
"id": "195cc1c6-db43-43a9-85be-3f4e90caec5b",
"archived": false,
"name": "CRM Contacts Demo",
"teamId": 34661,
"environmentId": "25e01f6b-08f8-4cea-ba11-892a0c0bbe59",
"helpContent": null,
"privateKeyId": 4931,
"legacyWebhookURL": null,
"createdById": 30180,
"createdAt": "2022-05-20T17:28:58.000Z",
"updatedAt": "2022-06-21T19:23:01.000Z",
"deleted": false,
"deletedById": null,
"deletedAt": null
}
}
GET
Bulk delete uploads
Endpoint: /delete/batches
Query Params:
Name | Required | Type | Value | Description |
---|---|---|---|---|
olderThanQuantity | true | string | ||
olderThanUnit | true | string | Enum: minute , hour , day , week , month | |
sendEmail | true | string | Enum: true , false | |
teamId | true | string |
Example Reponse:
- Types
- JSON
interface Response {
success: true;
}
{
"success": true
}
GET
List Workspace uploads
Endpoint: /rest/batches
Query Params:
Name | Required | Type | Value | Description |
---|---|---|---|---|
endUserId | string | Valid endUserId for the Workspace | ||
environmentId | string | Valid environmentId for the Workspace | ||
licenseKey | true | string | A valid licenseKey for the Workspace | |
search | string | Searches fileName , originalFile , memo | ||
skip | number | 0 (default) | The rows to skip before listing | |
take | number | 50 (default) | The maximum number of rows to return | |
workspaceId | string | Valid workspaceId for the Workspace |
Example Reponse:
- Types
- JSON
interface Response {
pagination: {
currentPage: number;
limit: number;
offset: number;
onPage: number;
nextOffset: number;
totalCount: number;
pageCount: number;
};
data: Array<Batch>;
}
{
"pagination": {
"currentPage": 1,
"limit": 50,
"offset": 0,
"onPage": 50,
"nextOffset": 50,
"totalCount": 161,
"pageCount": 4
},
"data": [
{
"id": "04f899aa-d802-44e3-b83a-2d9n7fa8407d",
"licenseId": 32335,
"teamId": 34661,
"environmentId": "25e01f6b-08f8-4cea-ba11-892a0c0bbe59",
"workspaceId": "b85bf6f6-866a-4e03-a3ae-7dc28bpba4e1",
"schemaId": 147732,
"embedId": null,
"endUserId": "4dacf193-7bb6-4402-b789-3f005b01588d",
"filename": "license-0368488c-db40-4cb4-b949-63547207b58b/batch-a4f899aa-d802-44e3-b83a-2d9e7fa8407d/upload-f99356ca-d9f1-4ad0-ae87-763156f4235d.csv",
"source": null,
"memo": null,
"validatedIn": null,
"originalFile": "20+_CRM_Contacts.csv",
"manual": false,
"managed": false,
"countRows": 22,
"countRowsInvalid": null,
"countRowsAccepted": null,
"countColumns": null,
"countColumnsMatched": null,
"headersRaw": null,
"headersMatched": [
{
"index": 0,
"letter": "",
"value": "Status",
"matched_key": "dealStatus"
},
{
"index": 1,
"letter": "",
"value": "Date of Birth",
"matched_key": "date"
},
{
"index": 2,
"letter": "",
"value": "Zip",
"matched_key": "postalCode"
},
{
"index": 3,
"letter": "",
"value": "Country",
"matched_key": "country"
},
{
"index": 4,
"letter": "",
"value": "Phone",
"matched_key": "phone"
},
{
"index": 5,
"letter": "",
"value": "Subscribed",
"matched_key": "optIn"
},
{
"index": 6,
"letter": "",
"value": "Email",
"matched_key": "email"
},
{
"index": 7,
"letter": "",
"value": "last",
"matched_key": "lastName"
},
{
"index": 8,
"letter": "",
"value": "first",
"matched_key": "firstName"
}
],
"status": "matchComplete",
"type": 2,
"targetSchema": null,
"parsingConfig": null,
"importedFromUrl": null,
"headerHash": null,
"failureReason": null,
"matchedAt": null,
"submittedAt": null,
"failedAt": null,
"handledAt": null,
"writeAccessKey": "db7b5565-f685-4a4d-9dff-c5510c9ad69c",
"legacySettingsId": null,
"legacyWebhookUrl": null,
"legacyHasFieldHooks": null,
"legacyHasRecordHooks": null,
"createdAt": "2022-06-25T02:24:37.000Z",
"updatedAt": "2022-06-25T02:24:59.000Z",
"devMode": false,
"deleted": false
}
]
}
GET
File upload meta data
Endpoint: /rest/batch/:batchId
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
batchId | true | string | A valid UUID |
Example Reponse:
- Types
- JSON
interface Response extends Batch {
__schema__: Schema;
__embed__: Embed;
__endUser__: EndUser;
__has_endUser__: boolean;
}
{
"id": "be2f075c-edef-4c8e-a3e5-b27e6587f731",
"licenseId": 32335,
"teamId": 34661,
"environmentId": "25e01f6b-08f8-4cea-ba11-892a0c0bbe59",
"workspaceId": "9712ca31-cd2b-4c48-9595-90e99e2858b9",
"schemaId": 150088,
"embedId": "295cc1c6-db43-43a9-85be-3f4e90caec5b",
"endUserId": "774ea422-8686-4de6-b17b-e454cc3e07b7",
"filename": "license-0368488c-db40-4cb4-b949-63547207b58b/batch-ce2f075c-eaef-4c8e-a3e5-b27e6587f731/upload-177be07b-ea10-4bec-91a7-9a0cb08a76fa.csv",
"source": null,
"memo": null,
"validatedIn": null,
"originalFile": "20+_CRM_Contacts.csv",
"manual": false,
"managed": false,
"countRows": 22,
"countRowsInvalid": null,
"countRowsAccepted": null,
"countColumns": null,
"countColumnsMatched": null,
"headersRaw": null,
"headersMatched": [
{
"index": 0,
"letter": "",
"value": "Status",
"matched_key": "dealStatus"
},
{
"index": 1,
"letter": "",
"value": "Date of Birth",
"matched_key": "date"
},
{
"index": 2,
"letter": "",
"value": "Zip",
"matched_key": "postalCode"
},
{
"index": 3,
"letter": "",
"value": "Country",
"matched_key": "country"
},
{
"index": 4,
"letter": "",
"value": "Phone",
"matched_key": "phone"
},
{
"index": 5,
"letter": "",
"value": "Subscribed",
"matched_key": "optIn"
},
{
"index": 6,
"letter": "",
"value": "Email",
"matched_key": "email"
},
{
"index": 7,
"letter": "",
"value": "last",
"matched_key": "lastName"
},
{
"index": 8,
"letter": "",
"value": "first",
"matched_key": "firstName"
}
],
"status": "submitted",
"type": 2,
"targetSchema": null,
"parsingConfig": null,
"importedFromUrl": "https://zmkcjc.csb.app/",
"headerHash": null,
"failureReason": null,
"matchedAt": null,
"submittedAt": "2022-06-22T14:41:58.000Z",
"failedAt": null,
"handledAt": null,
"writeAccessKey": "97f71958-2073-415e-9079-c179ed27607e",
"legacySettingsId": null,
"legacyWebhookUrl": null,
"legacyHasFieldHooks": null,
"legacyHasRecordHooks": null,
"createdAt": "2022-06-22T14:32:23.000Z",
"updatedAt": "2022-06-22T14:41:58.000Z",
"devMode": false,
"deleted": false,
"__schema__": {
"id": 150088,
"name": "CRM Contacts Demo",
"ancestorId": 149805,
"variantOfId": null,
"rootId": 139270,
"version": 111,
"archived": true,
"teamId": 34661,
"environmentId": "25e01f6b-08f8-4cea-ba11-892a0c0bbe59",
"createdById": 30180,
"jsonSchema": {
"schema": {
"properties": {
"firstName": {
"type": "string",
"label": "First Name"
},
"lastName": {
"type": "string",
"label": "Last Name"
},
"email": {
"type": "string",
"label": "Email Address",
"format": "email"
},
"phone": {
"type": "string",
"label": "Phone Number",
"format": "phone"
},
"date": {
"type": "string",
"label": "Date"
},
"country": {
"type": "string",
"label": "Country"
},
"postalCode": {
"type": "string",
"label": "Postal Code"
},
"optIn": {
"type": "boolean",
"label": "Opt In"
},
"dealStatus": {
"type": "string",
"label": "Deal Status",
"enumLabel": [
"Prospecting",
"Discovery",
"Proposal",
"Negotiation",
"Closed Won",
"Closed Lost"
],
"enum": [
"Prospecting",
"Discovery",
"Proposal",
"Negotiation",
"Closed Won",
"Closed Lost"
]
}
},
"type": "object",
"required": ["lastName"],
"unique": ["email"]
}
},
"importCount": 0,
"fieldCount": 9,
"createdAt": "2022-06-18T20:33:07.000Z",
"updatedAt": "2022-06-24T17:42:49.000Z",
"linkedSchemaIds": [],
"previewFieldKey": null
},
"__embed__": {
"id": "395cc1c6-db43-43a9-85be-3f4e90caec5b",
"archived": false,
"name": "CRM Contacts Demo",
"teamId": 34661,
"environmentId": "25e01f6b-08f8-4cea-ba11-892a0c0bbe59",
"helpContent": null,
"privateKeyId": 4931,
"legacyWebhookURL": null,
"createdById": 30180,
"createdAt": "2022-05-20T17:28:58.000Z",
"updatedAt": "2022-06-21T19:23:01.000Z",
"deleted": false,
"deletedById": null,
"deletedAt": null
},
"__endUser__": {
"id": "874ea422-8686-4de6-b17b-e454cc3e07b7",
"userId": "42",
"name": "Hans Hoffman",
"email": "hans@flatfile.io",
"companyId": "4242",
"companyName": "Flatfile",
"teamId": 34661,
"createdAt": "2022-06-16T18:31:27.000Z",
"updatedAt": "2022-06-24T18:12:15.000Z"
},
"__has_endUser__": true
}
GET
Sheet name for file upload
Endpoint: /upload/:uploadId/dataSources
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
uploadId | true | string | A valid UUID |
uploadId
can be found in thefilename
string:"license-53674a8c-db40-4cb4-b949-63547207b58b/batch-89fm0375-2760-4bb0-8359-6f62ba35c597/upload-dbf6f99c-851c-4aea-994c-97e0d203f178.xlsx"
. It's the UUID immediately following "upload-".
Query Params:
Name | Required | Type | Value | Description |
---|---|---|---|---|
licenseKey | string | A valid licenseKey for the Workspace |
Example Response:
- Types
- JSON
interface Response {
uploadId: UUID;
index: number;
label: string;
rowCount: number;
columnCount: number;
hasError: boolean;
errorMsg: string;
errorType: number;
path: Nullable<string>;
}
[
{
"uploadId": "cdc6f99c-851c-4aea-994c-97e0d203f178",
"index": 0,
"label": "sheet1",
"rowCount": 1,
"columnCount": 0,
"hasError": false,
"errorMsg": "",
"errorType": 0,
"path": null
}
]
GET
Records for file upload
Endpoint: /rest/batch/:batchId/rows
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
batchId | true | string | A valid UUID |
Query Params:
Name | Required | Type | Value | Description |
---|---|---|---|---|
createdAtEndDate | string | ISO 8601 | The maximum createdAt date to return | |
createdAtStartDate | string | ISO 8601 | The minimum createdAt date to return | |
deleted | string | Enum: true , t , y , false , f , n | Return only deleted rows | |
skip | number | 0 (default) | The rows to skip before listing | |
take | number | 50 (default) | The maximum number of rows to return | |
updatedAtEndDate | string | ISO 8601 | The maximum updatedAt date to return | |
updatedAtStartDate | string | ISO 8601 | The minimum updatedAt date to return | |
valid | string | Enum: true , t , y , false , f , n | Return only valid rows |
Example Reponse:
- Types
- JSON
interface Response {
pagination: {
currenPage: number;
limit: number;
offset: number;
onPage: number;
totalCount: number;
pageCount: number;
};
data: Array<{
id: UUID;
mapped: Record<string, any>;
}>;
}
{
"pagination": {
"currentPage": 1,
"limit": 50,
"offset": 0,
"onPage": 2,
"totalCount": 2,
"pageCount": 1
},
"data": [
{
"id": "7da9647a-bc1c-4823-bbd4-1f68ad6cd05e",
"mapped": {
"firstName": "GABBIE",
"lastName": "Meader",
"email": "gmeader@flatfile.com",
"phone": "",
"date": "",
"country": "US",
"postalCode": "93715",
"optIn": false,
"dealStatus": "Discovery"
}
},
{
"id": "8a57f0b8-9737-4e42-809c-45fdcfdc5c61",
"mapped": {
"firstName": "LYNETT",
"lastName": "Phizackerly",
"email": "lphizackerly1@cmu.edu",
"phone": "8178755582",
"date": "1991-12-17",
"country": "CA",
"postalCode": "J6R",
"optIn": false,
"dealStatus": "Prospecting"
}
}
]
}
POST
Upload to Workspace sheet
Endpoint: /workspace/:workspaceId/sheet/:sheetId/data
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
sheetId | true | string | A valid UUID | |
workspaceId | true | string | A valid UUID |
Request Body:
- Types
- JSON
interface Response {
data: Array<Record<string, any>>;
}
{
"data": [
{
"firstName": "foo",
"lastName": "bar",
"email": "foo@bar.com",
"phone": null,
"date": null,
"country": null,
"postalCode": null,
"optIn": null,
"dealStatus": null
}
]
}
Example Response:
- Types
- JSON
interface Response {
rows: Array<{
_id: number;
cells: Record<
string,
{
value: Nullable<any>;
}
>;
externalId: Nullable<string>;
validations: Array<{
key: string;
type: "type" | "value";
error: string;
message: string;
}>;
status: RecordStatus;
}>;
totalRows: number;
accepted: number;
dismissed: number;
filtered: number;
counts: {
valid: number;
invalid: number;
merged: number;
};
requestId: string;
}
{
"rows": [
{
"_id": 24,
"cells": {
"firstName": {
"value": null
},
"lastName": {
"value": null
},
"email": {
"value": null
},
"phone": {
"value": null
},
"date": {
"value": null
},
"country": {
"value": null
},
"postalCode": {
"value": null
},
"optIn": {
"value": null
},
"dealStatus": {
"value": null
}
},
"validations": [
{
"key": "lastName",
"type": "value",
"error": "error",
"message": "Required value"
},
{
"key": "email",
"type": "type",
"error": "warn",
"message": "Must have either phone or email"
},
{
"key": "phone",
"type": "type",
"error": "warn",
"message": "Must have either phone or email"
}
],
"status": "review",
"externalId": null
}
]
}
GET
Fetch Workspace sheet records
Endpoint: /workspace/:workspaceId/sheet/:sheetId/records
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
sheetId | true | string | A valid UUID | |
workspaceId | true | string | A valid UUID |
Query Params:
Name | Required | Type | Value | Description |
---|---|---|---|---|
filter | string | Enum: review , dismissed , accepted | Return only the filtered rows | |
mergeId | string | |||
nested | boolean | |||
recordIds | string[] | |||
skip | number | 0 (default) | The rows to skip before listing | |
take | number | 50 (default) | The maximum number of rows to return | |
valid | string | Enum: true , t , y , false , f , n | Return only valid rows |
Example Response:
- Types
- JSON
interface Response {
rows: Array<{
_id: number;
cells: Record<
string,
{
value: any;
}
>;
validations: Array<{
error: string;
key: string;
message: string;
}>;
status: RecordStatus;
externalId: Nullable<string>;
}>;
totalRows: number;
accepted: number;
dismissed: number;
filtered: number;
counts: {
valid: number;
invalid: number;
merged: number;
};
}
{
"rows": [
{
"_id": 1,
"cells": {
"firstName": {
"value": "Gabbie"
},
"lastName": {
"value": "Meader"
},
"email": {
"value": "gmeader@flatfile.com"
},
"phone": {
"value": ""
},
"date": {
"value": ""
},
"country": {
"value": "US"
},
"postalCode": {
"value": "93715"
},
"optIn": {
"value": false
},
"dealStatus": {
"value": "deal"
}
},
"validations": [
{
"key": "country",
"type": "type",
"error": "info",
"message": "Country was automatically formatted"
},
{
"key": "dealStatus",
"type": "value",
"error": "error",
"message": "should be equal to one of the allowed values"
}
],
"status": "review",
"externalId": null
}
],
"totalRows": 1,
"accepted": 0,
"dismissed": 21,
"filtered": 1,
"counts": {
"valid": 0,
"invalid": 1,
"merged": 0
}
}
GET
List team Workspaces
Endpoint: /rest/teams/:teamId/workspaces
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
teamId | true | string |
Query Params:
Name | Required | Type | Value | Description |
---|---|---|---|---|
environmentId | string | Valid environmentId for the Workspace | ||
skip | number | 0 (default) | The rows to skip before listing | |
take | number | 50 (default) | The maximum number of rows to return |
Example Reponse:
- Types
- JSON
interface Response {
pagination: {
currenPage: number;
limit: number;
offset: number;
onPage: number;
totalCount: number;
pageCount: number;
};
data: Array<Workspace>;
}
{
"pagination": {
"currentPage": 1,
"limit": 50,
"offset": 0,
"onPage": 2,
"totalCount": 2,
"pageCount": 1
},
"data": [
{
"id": "935bf6f6-866a-4e03-a3ae-7dc28bcba4e1",
"environmentId": "25e01f6b-08f8-4cea-ba11-892a0c00be59",
"primaryWorkbookId": "0b059cf8-7m7c-426b-9064-5cf047ec0d15",
"secureEnvId": null,
"name": "CRM Contacts Demo",
"slug": null,
"sessionKey": null,
"status": 0,
"type": 0,
"dataEngine": 1,
"createdAt": "2022-05-09T22:03:49.000Z",
"updatedAt": "2022-06-09T17:38:09.000Z",
"deleted": null
},
{
"id": "66d84cf2-0667-4dp8-8c39-ba031323c250",
"environmentId": "25e0186b-08f8-4ceb-ba11-892a0c0bbe59",
"primaryWorkbookId": "96d5a33d-0766-4fja-8810-1be1f3232cef",
"secureEnvId": null,
"name": "Special Demo",
"slug": null,
"sessionKey": null,
"status": 1,
"type": 0,
"dataEngine": 1,
"createdAt": "2022-06-02T16:03:39.000Z",
"updatedAt": "2022-06-02T16:03:39.000Z",
"deleted": null
}
]
}
GET
Detail Workspace
Endpoint: /rest/workspace/:workspaceId
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
workspaceId | true | string | A valid UUID |
Example Reponse:
- Types
- JSON
interface Response extends Workspace {}
{
"id": "b35bf6f6-8669-4e03-a3ae-7dc28bcba4e1",
"environmentId": "26e01f6b-08f8-4cea-ba11-892a0c0bbe59",
"primaryWorkbookId": "0a059cf8-7e7c-426b-9064-5cfd47ec0d15",
"secureEnvId": null,
"name": "CRM Contacts Demo",
"slug": null,
"sessionKey": null,
"status": 0,
"type": 0,
"dataEngine": 1,
"createdAt": "2022-05-09T22:03:49.000Z",
"updatedAt": "2022-06-09T17:38:09.000Z",
"deleted": null
}
POST
Invite Workspace collaborator
Endpoint: /rest/teams/:teamId/workspaces/:workspaceId/invitations
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
teamId | true | string | ||
workspaceId | true | string | A valid UUID |
Request Body:
Name | Required | Type | Value | Description |
---|---|---|---|---|
email | true | string | Email address of invited collaborator |
Example Reponse:
- Types
- JSON
interface Response {
success: boolean;
message?: string;
}
{
"success": true
}
GET
List Workspace invitations
Endpoint: /rest/teams/:teamId/workspaces/:workspaceId/invitations
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
teamId | true | string | ||
workspaceId | true | string | A valid UUID |
Example Reponse:
- Types
- JSON
interface Invitation {
deleted: boolean;
expired: boolean;
id: UUID;
workspaceId: UUID;
adminTeamId: number;
organizationId: UUID;
name: string;
email: string;
token: UUID;
invitedById: Nullable<number>;
createdAt: ISO_8601;
updatedAt: ISO_8601;
activatedAt: ISO_8601;
deletedAt: Nullable<ISO_8601>;
}
type Response = Array<Invitation>;
[
{
"deleted": false,
"expired": true,
"id": "83237ad1-531f-4d61-b60a-807370d4d17e",
"workspaceId": "a55bf6f6-866a-4e03-a3ae-7dc28bcba4e1",
"adminTeamId": 00661,
"organizationId": "9b1d3b66-94bf-40f4-a9cd-53dae5a563e3",
"name": "Foobar",
"email": "foobar@foobar.com",
"token": "596ac3db-3a52-40e0-943f-d2dc896b7a83",
"invitedById": null,
"createdAt": "2022-07-15T14:48:29.000Z",
"updatedAt": "2022-07-15T14:48:29.000Z",
"activatedAt": "2022-07-15T14:48:29.000Z",
"deletedAt": null
}
]
GET
List Workspace collaborators
Endpoint: /rest/teams/:teamId/workspaces/:workspaceId/collaborators
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
teamId | true | string | ||
workspaceId | true | string | A valid UUID |
Example Reponse:
- Types
- JSON
interface Collaborator {
id: number;
name: string;
oauthGitHubId: Nullable<string>;
email: string;
githubUsername: Nullable<string>;
trialEndsAt: Nullable<ISO_8601>;
lastLoginAt: Nullable<ISO_8601>;
createdAt: ISO_8601;
updatedAt: ISO_8601;
}
type Response = Array<Collaborator>;
[
{
"id": 32290,
"name": "FooBar",
"oauthGitHubId": null,
"email": "foo@bar.com",
"githubUsername": null,
"trialEndsAt": null,
"lastLoginAt": null,
"createdAt": "2022-07-19T18:08:35.000Z",
"updatedAt": "2022-07-19T18:08:35.000Z"
}
]
DELETE
Revoke Workspace invitation
Endpoint: /rest/teams/:teamId/workspaces/:workspaceId/invitations
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
teamId | true | string | ||
workspaceId | true | string | A valid UUID |
Request Body:
Name | Required | Type | Value | Description |
---|---|---|---|---|
email | true | string | Email address of invited collaborator |
Example Reponse:
- Types
- JSON
interface Response {
success: boolean;
message?: string;
}
{
"success": true
}
DELETE
Remove Workspace collaborator
Endpoint: /rest/teams/:teamId/workspaces/:workspaceId/collaborators/:userId
Path Variables:
Name | Required | Type | Value | Description |
---|---|---|---|---|
teamId | true | string | ||
userId | true | string | ||
workspaceId | true | string | A valid UUID |
Request Body:
Name | Required | Type | Value | Description |
---|---|---|---|---|
email | true | string | Email address of collaborator |
Example Reponse:
- Types
- JSON
interface Response {
success: boolean;
message?: string;
}
{
"success": true
}
Common types
type UUID = string; /* 021f68c8-68f6-4910-8d07-9c505683b1d4 */
type ISO_8601 = string; /* 2022-05-20T17:28:58.000Z */
type BatchStatus =
/* anywhere from embed is opened to file started upload but did not finish / was not parsed */
| "created"
/* anywhere from file was uploaded and parsed through importer saw header selection but did not proceed */
| "header_confirmed"
/* anywhere from importer continued past header selection to some mapping done (even by us) */
| "imported"
/* anywhere from we automapped some fields to the user mapped fields but did not continue to review */
| "matched"
/* anywhere from importer continued after mapping (including loading time) until the data was submitted in review */
| "partial_matched"
/* data was submitted */
| "submitted";
type RecordStatus =
/* a record still in review */
| "review"
/* a record explicity set to be dismissed */
| "dismissed"
/* a record explicity set to be accepted */
| "accepted";
type BatchType =
/* Legacy */
| 0
/* Throw away -- do not use */
| 1
/* File Upload */
| 2
/* Robot */
| 3;
type Nullable<A> = A | null; /* any value of type A or null */
type UploadSource = 1; /* SFTP */
interface Embed {
id: UUID;
archived: boolean;
name: string;
teamId: number;
environmentId: UUID;
helpContent: Nullable<string>;
privateKeyId: number;
legacyWebhookURL: Nullable<string>;
createdById: number;
createdAt: ISO_8601;
updatedAt: ISO_8601;
deleted: boolean;
deletedById: Nullable<number>;
deletedAt: Nullable<ISO_8601>;
}
interface Workspace {
id: UUID;
environmentId: UUID;
primaryWorkbookId: UUID;
secureEnvId: Nullable<UUID>;
name: string;
slug: Nullable<string>;
sessionKey: Nullable<string>;
status: number;
type: number;
dataEngine: number;
createdAt: ISO_8601;
updatedAt: ISO_8601;
deleted: Nullable<boolean>;
}
interface Schema {
id: number;
name: string;
ancestorId: number;
variantOfId: Nullable<number>;
rootId: number;
version: number;
archived: boolean;
teamId: number;
environmentId: UUID;
createdById: number;
jsonSchema: {
schema: {
properties: Record<
string,
{
default?: any;
enum?: Array<string>;
enumLabel?: Array<string>;
format?: string;
label: string;
maximum?: number;
minimum?: number;
regexp?: {
pattern: string;
flags: string;
ignoreBlanks: boolean;
};
schemaId?: string;
type: string;
visibility?: Partial<Record<"export" | "mapping" | "review", boolean>>;
}
>;
type: "object";
required: Array<string>;
unique: Array<string>;
};
};
importCount: number;
fieldCount: number;
createdAt: ISO_8601;
updatedAt: ISO_8601;
linkedSchemaIds: Array<number>;
previewFieldKey: Nullable<string>;
}
interface EndUser {
id: UUID;
userId: string;
name: string;
email: string;
companyId: string;
companyName: string;
teamId: number;
createdAt: Nullable<ISO_8601>;
updatedAt: Nullable<ISO_8601>;
}
interface Batch {
id: UUID;
licenseId: number;
teamId: number;
environmentId: UUID;
workspaceId: UUID;
schemaId: number;
embedId: UUID;
endUserId: UUID;
filename: Nullable<string>;
source: Nullable<UploadSource>;
memo: Nullable<string>;
validatedIn: Nullable<string>;
originalFile: Nullable<string>;
manual: Nullable<boolean>;
managed: Nullable<boolean>;
countRows: Nullable<number>;
countRowsInvalid: Nullable<number>;
countRowsAccepted: Nullable<number>;
countColumns: Nullable<number>;
countColumnsMatched: Nullable<number>;
headersRaw: Nullable<
Array<{
index: number;
letter: string;
value: string;
}>
>;
headersMatched: Nullable<
Array<{
index: number;
letter: string;
value: string;
matched_key: string;
}>
>;
status: Nullable<BatchStatus>;
type: BatchType;
targetSchema: null;
parsingConfig: null;
importedFromUrl: string;
headerHash: Nullable<string>;
failureReason: Nullable<string>;
matchedAt: Nullable<ISO_8601>;
submittedAt: Nullable<ISO_8601>;
failedAt: Nullable<ISO_8601>;
handledAt: Nullable<ISO_8601>;
writeAccessKey: Nullable<UUID>;
legacySettingsId: Nullable<UUID>;
legacyWebhookUrl: Nullable<string>;
legacyHasFieldHooks: Nullable<boolean>;
legacyHasRecordHooks: Nullable<boolean>;
createdAt: ISO_8601;
updatedAt: Nullable<ISO_8601>;
devMode: boolean;
deleted: boolean;
}